CSS背景属性创建动态边栏和动画
2024-10-16
情景:创建动态边栏
假设你在构建一个响应式的网站,需要在滚动到页面的某一部分时改变文本的颜色。这里我们使用背景属性来实现这一目标。
步骤1: 理解 CSS 属性
我们需要理解不同的 CSS 属性如何在背景中进行应用,这包括:
- background-color: 设置主背景色。
- background-image: 允许我们将图像作为背景使用。
- background-position: 控制背景图像在元素上的位置。
- background-repeat: 确定背景图像是否在容器的边界上重复。
- background-size: 指明背景图像在容器中的缩放方式。
步骤2: CSS 背景属性
让我们进入实现示例。这里是一个使用这些属性创建一个动态边栏的例子:
/* 设置主颜色 */
footer {
background-color: #f0f0f0;
}
/* 设置固定高度的边栏,以便它不会随着窗口大小的变化而变化 */
footer {
height: 50px;
}
/* 点缀文本内容以更改其颜色在滚动过程中*/
.footer-text {
position: relative;
color: white;
font-size: 16px;
text-align: center;
}
/* 使用固定背景图像来设置边栏的背景,并且使用 no-repeat 和 fixed 属性确保背景图像不进行缩放 */
.footer-text {
background-image: url('footer-background.png');
background-position: center;
background-repeat: no-repeat;
background-size: cover;
}
/* 创建一个滚动动画来改变文本的颜色,颜色从黑色变为白色和再变回黑色 */
@keyframes colorChange {
0% { color: black; }
50% { color: white; }
100% { color: black; }
}
/* 将动画应用于文本内容上,并使其持续3秒钟 */
.footer-text {
animation-name: colorChange;
animation-duration: 3s;
}
解释:
-
footer
样式 是用于针对边栏元素的应用。 -
background-color
属性设置了一定基色,可以进行定制。 - 创建一个名为
colorChange
的关键帧动画,该动画将文本的颜色从黑色变为白色然后变回黑色。这个通过animation-name
这个选项在.footer-text
上应用,并且它会持续 3 秒钟进行变换。
步骤3: 使用 Flexbox 或 Grid
对于更精细的布局控制和响应性,考虑使用 CSS flexbox 或 grid。这里是一个例子:
/* 使用 flexbox 进行布局 */
footer {
display: flex;
align-items: center; /* 中心对齐项目在垂直方向上 */
justify-content: space-between; /* 确保项目在水平方向上等距排列 */
}
.footer-text {
font-size: 20px;
color: white;
background-image: url('footer-background.png');
background-position: center;
background-repeat: no-repeat;
height: 45px;
margin: auto;
}
@keyframes colorChangeFlexbox {
0% { background-color: black; }
50% { background-color: white; }
100% { background-color: black; }
}
.footer-text {
animation-name: colorChangeFlexbox;
animation-duration: 3s;
}
总结
通过掌握 CSS 背景属性,你可以创建一系列动态且引人注目的效果。从简单的颜色更改到更复杂的动画,理解这些技术使你能够构建响应式和令人满意的用户体验。
请记住,在不同浏览器和设备上测试您的代码以确保兼容性和性能优化是非常重要的。 当然,我理解你的担忧,特别是在不同的浏览器和设备上测试HTML和CSS以确保其兼容性以及性能优化的重要性。这是一个很好的建议,因为每个用户可能使用不同的浏览器或设备访问你的网站。
在HTML和CSS代码中包含一些关键点可以帮助你在不同平台上实现更好的表现:
-
响应式设计:使用CSS媒体查询(Media Queries)来确保你的布局适应各种屏幕尺寸。例如:
/* 响应式背景图像 */ @media (max-width: 600px) { footer { background-image: url('footer-background-mobile.png'); } } @media (min-width: 768px) and (max-width: 1200px) { footer { background-image: url('footer-background-desktop.png'); } } /* 响应式文本颜色变化 */ @keyframes colorChangeFlexbox { 0% { font-weight: bold; } 50% { font-weight: normal; } 100% { font-weight: bold; } } .footer-text { animation-name: colorChangeFlexbox; animation-duration: 3s; }
-
媒体查询:在不同的屏幕尺寸下为背景图像和文本颜色设置不同的样式,从而确保它们适应各种设备。
/* 响应式背景图像 */ footer { background-image: url('footer-background-desktop.png'); } @media (max-width: 768px) and (min-width: 480px) { footer { height: 35px; font-size: 14px; } } /* 响应式文本颜色变化 */ .footer-text { animation-name: colorChangeFlexbox; animation-duration: 3s; }
-
JavaScript和jQuery:如果你需要更复杂的动画或交互,可以使用JavaScript或jQuery。确保这些脚本是经过测试的,并在所有目标浏览器中都正常工作。
// 基于用户滚动位置改变文本颜色的JavaScript示例 function changeTextColor() { var header = document.querySelector('header'); if (window.scrollY > 10) { header.className += ' scroll'; } else { header.className = header.className.replace(/ scroll/, ''); } } // 添加滚动检测事件到body元素 window.addEventListener('scroll', changeTextColor);
-
使用在线工具和库:例如,可以使用工具如BrowserStack(浏览器堆栈)来模拟不同的设备和浏览器环境。也可以查看MDN文档或开发者社区中的教程,以获取更具体的解决方案。
在开发过程中,确保代码兼容性和性能优化对于最终用户的体验至关重要。通过仔细测试和调整代码,你可以确保你的网站在各种情况下都能提供良好的用户体验。
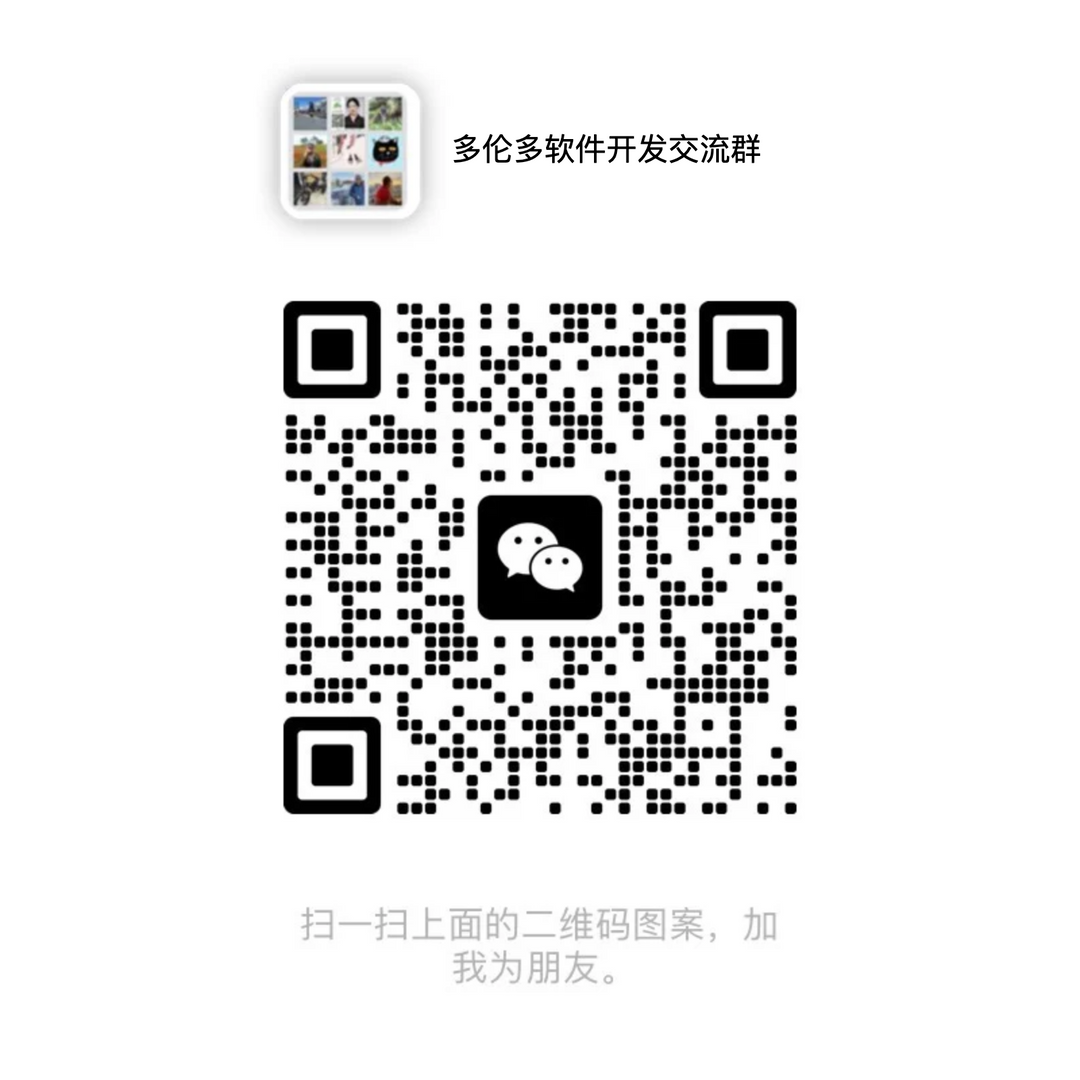