React、Angular和Vue.js前端框架组件样式指南
2024-10-18
作为前端开发人员,掌握如何有效地为组件进行样式设计是至关重要的。本指南将专注于三种流行的React前端框架:React、Angular和Vue.js,并提供一些示例来帮助解释概念。
让我们从一个场景开始:
假设您正在构建一个简单博客应用程序,使用这些框架。前端应该具有响应性、可访问性和易于使用的特性,适用于所有设备和能力的用户。该应用将显示分类、搜索结果和帖子的不同部分。每个部分都会有自己的样式。
- React.js
React是目前最流行的前端库之一,由Facebook开发。它以其性能和灵活性而闻名。以下是一个简单的博客文章组件示例,其中包含一个带有按钮的标题:
import React from 'react';
const Post = () => {
return (
<div>
<h1>Post Title</h1>
<p>Description goes here...</p>
<button className="post-button">Read More</button>
</div>
);
};
export default Post;
为了为这个组件进行样式,您可以使用CSS in JavaScript(CSS-in-JS)或编写一个单独的样式表。
.post-button {
background-color: #3498db; /* 暗蓝板正色 */
border: none;
color: white;
padding: 10px 20px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 10px;
cursor: pointer;
width: 150px; /* 根据您的需求调整 */
transition: background-color 0.3s, border-color 0.3s, box-shadow 0.3s;
}
.post-button:hover {
background-color: #28a745; /* 绿灰色蓝 */
color: white;
}
这是一个示例,展示了如何为使用CSS in JavaScript或单独样式表进行样式。
- Angular
Angular是另一个流行的前端框架,用于开发大规模应用程序。它提供了比React更多的功能,如双向数据绑定、依赖注入和异步编程等。
import { Component } from '@angular/core';
@Component({
selector: 'app-post',
templateUrl: './post.component.html',
styleUrls: ['./post.component.css']
})
export class PostComponent {
post = {
title: 'Post Title',
description: 'Description goes here...'
};
readMore() {
console.log('Read more clicked');
}
}
<style>
.post-button {
background-color: #3498db; /* 暗蓝板正色 */
border: none;
color: white;
padding: 10px 20px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 10px;
cursor: pointer;
width: 150px; /* 根据您的需求调整 */
transition: background-color 0.3s, border-color 0.3s, box-shadow 0.3s;
}
.post-button:hover {
background-color: #28a745; /* 绿灰色蓝 */
color: white;
}
</style>
这是使用Angular框架的示例,展示如何为Vue组件进行样式。
- Vue.js
Vue是一个流行的前端库,用于构建单页应用程序和具有JavaScript的混合移动应用程序。它以其简洁、灵活性和易于使用而闻名。
<template>
<div>
<h1 v-if="showTitle">Post Title</h1>
<p v-if="showDescription">Description goes here...</p>
<button @click="readMore" class="post-button">Read More</button>
</div>
</template>
<script>
export default {
data() {
return {
showTitle: false,
showDescription: true
};
},
methods: {
readMore() {
this.showTitle = true;
this.showDescription = false;
}
}
}
</script>
<style>
.post-button {
background-color: #3498db; /* 暗蓝板正色 */
border: none;
color: white;
padding: 10px 20px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 10px;
cursor: pointer;
width: 150px; /* 根据您的需求调整 */
transition: background-color 0.3s, border-color 0.3s, box-shadow 0.3s;
}
.post-button:hover {
background-color: #28a745; /* 绿灰色蓝 */
color: white;
}
</style>
这是一个示例,展示了如何使用Vue的v-if指令和CSS in JavaScript进行样式。
总结一下,在为React组件设置样式时,重要的是要考虑可访问性、响应性和性能。您可以使用CSS-in-JS或单独的样式表来更好地组织和维护代码。有了这些示例,现在应该对使用不同前端框架为自己的组件进行样式设计有了良好的理解。 | Framework | Description | Example Component | CSS/Styles | | --- | --- | --- | --- | | React.js | One of the most popular front-end libraries, developed by Facebook. Known for its performance and flexibility. |
Post Title
Description goes here...
<post-button> Read More </post-button>
|
| React.js (CSS-in-JS) | An example using CSS in JavaScript. This allows for less maintenance since styles are managed within the component's code. | {this.state.title}
{this.state.description && ({this.state.description}
)}const postButton = () => { return ( <button className="post-button" > Read More </button> ) }
|
| Angular | A framework that includes features like two-way data binding, dependency injection and asynchronous programming. Provides more functionality than React. | <div [ngClass]="{'show-title': showTitle, 'hide-description': !showDescription}"> <h1 *ngIf="showTitle" class="post-title">Post Title <p *ngIf="showDescription" class="post-description">Description goes here... <button @click="readMore()" class="post-button">Read More export default class PostComponent { constructor() { this.showTitle = false; this.showDescription = true; } readMore() { this.showTitle = !this.showTitle; this.showDescription = !this.showDescription; } }
|
| Vue.js | A popular library for building single-page applications and hybrid mobile applications, known for its simplicity, flexibility, and ease of use. Known for v-if directives. | Description goes here...
<button @click="readMore()" class="post-button">Read More |<post-button> Read More </post-button>
|
| Vue.js (CSS-in-JS) | An example using CSS in JavaScript. This allows for better organization and maintenance of styles within the component's code. | {{ title }}
{{ description }}
<button @click="readMore()" class="post-button">Read Moreexport default Vue.component('app-post', { data() { return { showTitle: false, showDescription: true } }, methods: { readMore() { this.showTitle = !this.showTitle; this.showDescription = !this.showDescription; } } });
|
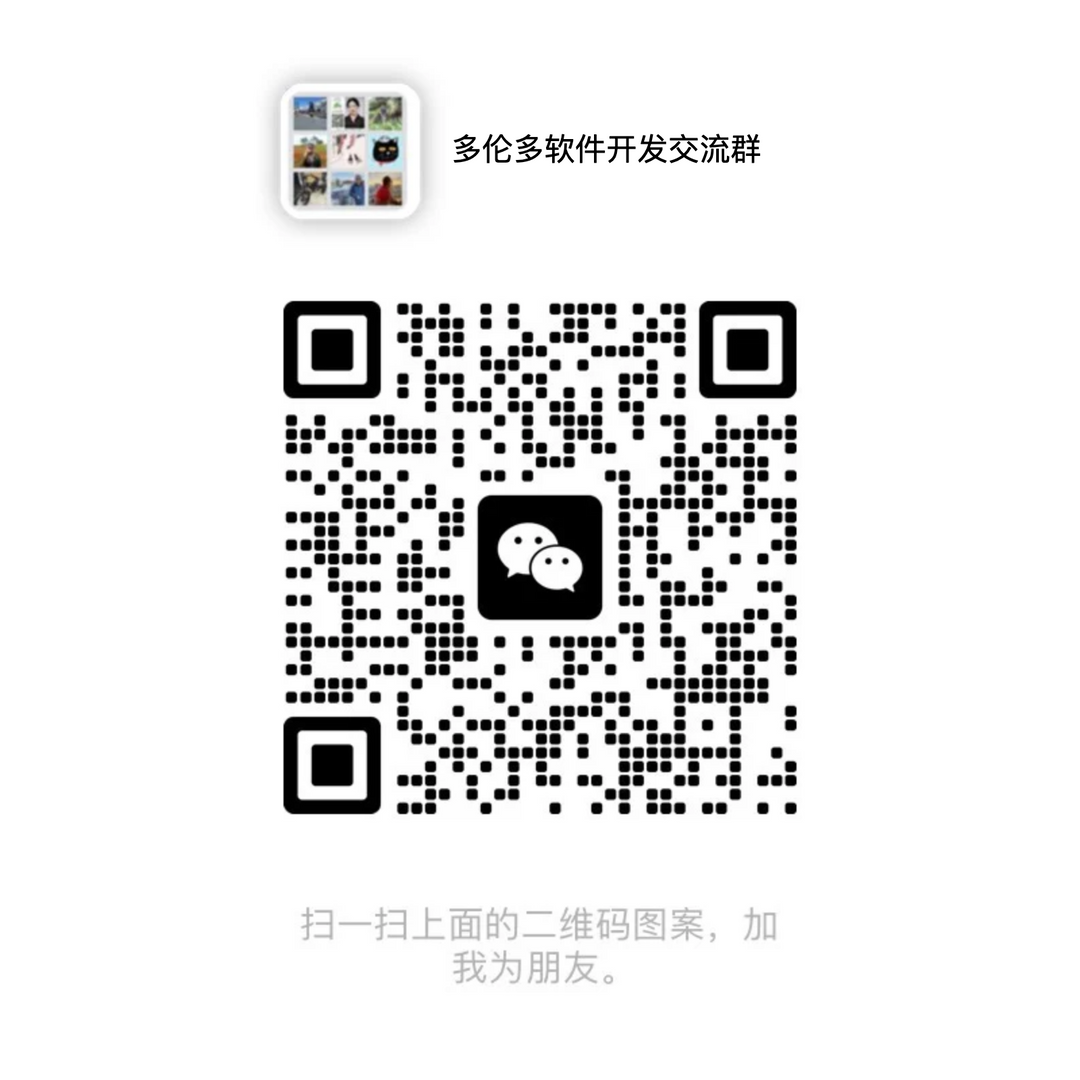